目的
Gtk.Entryには、①下部分に棒状のものが左右に往復するパルスや②前後にアイコンを表示させることが可能です。今回はその設定方法について見ていきます。
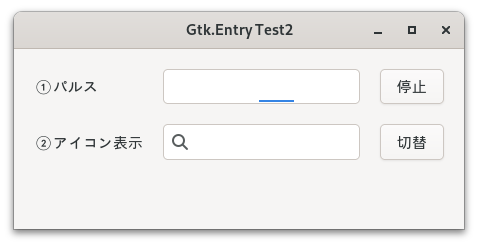
Gtk.Entryの設定
Gtk.Entryにパルスを表示する
Gtk.Entryにパルスを表示するには、①メソッドset_progress_pulse_step()でパルスの移動量を指定して、②progress_pulse()を実行する度にパルスを指定した移動量分だけ動かします。
サンプルプログラムでは、GLib.timeout_add()を使用して0.1秒間隔でprogress_pulse()を実行させています。
Gtk.Entryにアイコンを表示する
Gtk.Entryには、前側と後側にアイコンを表示することができます。アイコンの指定は、プロパティではprimary-icon-name
(前側)やsecondary-icon-name
(後側)で行い、メソッドではset_icon_from_icon_name()で行います。set_icon_from_icon_name(icon_pos, icon_name)のicon_posは、Gtk.EntryIconPositionで指定します。
使用するIconは、アプリケーション”Icon Browser”で探すことができます。
Gtk.Entryのサンプルプログラム②
以下のサンプルプログラムを実行すると、下図のような2組のGtk.LabelとGtk.Entry、Gtk.Buttonが表示されます。
①パルスと同じ並びのGtk.Button(ラベルが「実行」もしくは「停止」のもの)を押すと、パルスが動いたり(ラベル表示「実行」時)、停止したり(ラベル表示「停止」時)します。
②アイコンと同じ並びのGtk.Button(ラベルが「切替」のもの)を押すと、アイコンの表示位置がGtk.Entryの前から後ろもしくはその逆に移動します。
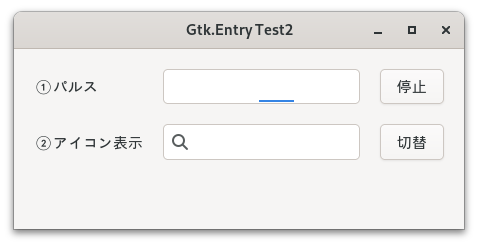
import gi
gi.require_version('Gtk', '4.0')
from gi.repository import Gtk, GLib
APPID = 'com.github.taniyoshima.g4_fblog_entry2'
class Gtk4TestTest(Gtk.Window):
def __init__(self, app):
Gtk.Window.__init__(
self, application=app, title='Gtk.Entry Test2',
default_width=450, default_height=180)
self.timeout_id = None
grid = Gtk.Grid(
margin_top=20, margin_bottom=20,
margin_start=20, margin_end=20,
row_spacing=20, column_spacing=20
)
label1 = Gtk.Label(label='①パルス', xalign=0.0)
label2 = Gtk.Label(label='②アイコン表示', xalign=0.0)
self.entry1 = Gtk.Entry()
self.entry2 = Gtk.Entry(primary_icon_name='system-search-symbolic')
button1 = Gtk.Button(label='実行')
button1.connect('clicked', self.on_button1_clicked)
button2 = Gtk.Button(label='切替')
button2.connect('clicked', self.on_button2_clicked)
grid.attach(label1, 0, 0, 1, 1)
grid.attach(label2, 0, 1, 1, 1)
grid.attach(self.entry1, 1, 0, 1, 1)
grid.attach(self.entry2, 1, 1, 1, 1)
grid.attach(button1, 2, 0, 1, 1)
grid.attach(button2, 2, 1, 1, 1)
self.set_child(grid)
def on_button1_clicked(self, button):
if button.get_label() == '実行':
self.entry1.set_progress_pulse_step(0.2)
self.timeout_id = GLib.timeout_add(100, self.do_pulse, None)
button.set_label('停止')
else:
GLib.source_remove(self.timeout_id)
self.timeout_id = None
self.entry1.set_progress_pulse_step(0)
button.set_label('実行')
def do_pulse(self, user_data):
self.entry1.progress_pulse()
return True
def on_button2_clicked(self, button):
if self.entry2.get_icon_name(Gtk.EntryIconPosition.PRIMARY) is None:
self.entry2.set_icon_from_icon_name(
Gtk.EntryIconPosition.PRIMARY, 'system-search-symbolic'
)
self.entry2.set_icon_from_icon_name(
Gtk.EntryIconPosition.SECONDARY, None
)
else:
self.entry2.set_icon_from_icon_name(
Gtk.EntryIconPosition.PRIMARY, None
)
self.entry2.set_icon_from_icon_name(
Gtk.EntryIconPosition.SECONDARY, "system-search-symbolic"
)
class Gtk4TestApp(Gtk.Application):
def __init__(self):
Gtk.Application.__init__(self, application_id=APPID)
def do_activate(self):
window = Gtk4TestTest(self)
window.present()
def main():
app = Gtk4TestApp()
app.run()
if __name__ == '__main__':
main()
27、28行目: Gtk.Entryの定義、両方ともインスタンス変数にしています。
self.entry2には、primary_icon_name=’system-search-symbolic’により、
頭に虫眼鏡のアイコンを付けています。
46〜55行目: button1(ラベルが「実行」もしくは「停止」のボタン)を押した場合の処理
button1のラベルが「実行」だった場合、
①パルスのステップを0.2にして、
②self.do_pulseを0.1秒周期で繰り返す作業(on_pulse())を開始、
③button1のラベルを「停止」にします。
button1のラベルが「停止」だった場合、
①self.timeout_idを使用して繰り返し作業を停止(除去)して、
②self.timeout_idをNoneにして、
③パルスのステップを0.2にして、
④button1のラベルを「実行」にします。
57〜59行目: 関数on_pluse: self.entry1.progress_pulse()を実行します。
※ 繰り返し作業をするには、return Trueが必須。
61〜75行目: button2を押した場合の処理
self.entry2の前側にアイコンがあるかを判定して、
①有る場合はアイコンを前から後ろに、
②無い場合はアイコンを後ろから前に移動します。