目的
Gtk.SpinButtonは、整数もしくは小数の値を表示するのに使用するものであり、数字をGtk.Entryに直接入力する代わりに、2つの矢印を押すことで表示された値を増減することができます。そのGtk.SpinButtonに表示する値の範囲や値、増減量は、Gtk.Adjustmentで指定します。
今回は、Gtk.SpinButtonの動作の指定方法及び、Gtk.Adjustmentの指定方法を見ていきます。
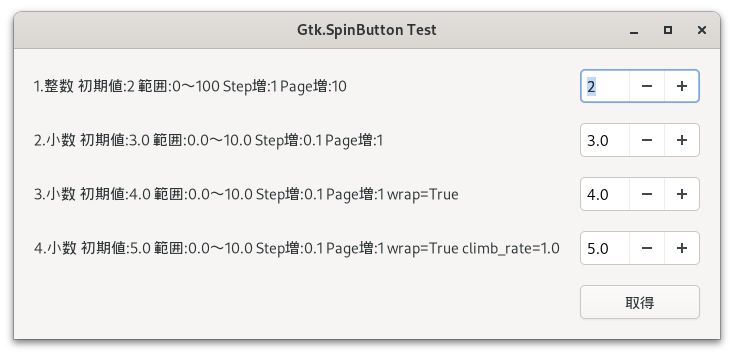
Gtk.SpinButtonの設定
Gtk.SpinButtonの設定は、プロパティやメソッドで指定します。Gtk.SpinButtonのプロパティは以下の表の通りであり、その他に継承したクラスのプロパティが使用できます。
名前 | 型 | 初期値 | 内容 |
---|---|---|---|
adjustment | Gtk.Adjustment | None | スピンボタンの値を保持するGtk.Adjustment |
climb-rate | float | 0.0 | ボタンまたはキーを長押しした場合の加速率。 大きいほどボタンを数字が早く変化する。 |
digits | int | 0 | 表示する小数点以下の桁数 |
numeric | bool | False | 数字以外の文字を無視するかどうかを指定する。 |
snap-to-ticks | bool | False | 誤った値を、ステップの増分に最も近い スピンボタンに自動的に変更するかどうかを指定する。 |
update_policy | Gtk. SpinButtonUpdatePolicy | Gtk .SpinButtonUpdatePolicy .ALWAYS | スピンボタンを常に更新するか、値が許容可能な場合にのみ更新するかを指定する。 |
value | float | 0.0 | スピンボタンの値。 |
wrap | bool | False | 上限(下限)に来た場合に、 下限(上限)の値に移動するかどうか |
Gtk.SpoinButtonUpdatePolicyには、以下の2つの値があり、どちらかを指定します。
値 | 内容 |
---|---|
Gtk.SpinButtonUpdatePolicy.ALWAYS | スピンボタンを更新すると、値が常に表示される。 ※ 既定値 |
Gtk.SpinButtonUpdatePolicy.IF_VALID | スピンボタンの更新した値が、スピンボタンの調整範囲内で有効な場合にのみ表示される。 |
Gtk.Adjustmentについて
Gtk.Adjustmentの設定は、プロパティやメソッドで行います。下の表は、Gtk.SpinButtonで使用するGtk.Adjustmentの設定をまとめたものです。
名前 | 型 | 初期値 | 内容 |
---|---|---|---|
lower | float | 0.0 | Gtk.Adjuctmentの下限値 |
page-increment | float | 0.0 | 「Page Up」「Page Down」キーを 押した場合の増減量 |
step-increment | float | 0.0 | ボタンを押した時の増減量 |
upper | float | 0.0 | Gtk.Adjuctmentの上限値 |
value | float | 0.0 | Gtk.Adjustmentの値 |
Gtk.SpinButtonに表示する値の範囲や値、増減量は、Gtk.Adjustmentに条件を指定して、それをプロパティadjustmentやメソッドset_adjustment()で指定することで設定します。
# Gtk.Adjustmentの定義
adjustment = Gtk.Adjustment(
lower=0, upper=100, value=2,
step_increment=1, page_increment=10
)
# Gtk.SpinButtonのadjustmentの指定
self.spinbutton1 = Gtk.SpinButton(
adjustment=adjustment
)
Gtk.SpinButtonのサンプルプログラム
以下のサンプルプログラムを実行すると、下図のような5つのGtk.LabelとGtk.SpinButton及びGtk.Buttonが表示されます。Gtk.SpinButtonの設定は、その左横のGtk.Labelに記入した通りであり、Gtk.Buttonを押すと、ターミナルにその時のGtk.SpinButtonの値が表示されます。
Pythonでは、Floatの値は正確でないため(例: 3.9が3.900000000000001になる)ターミナルに表示する時には、取得した値に対して桁数の指定を行っています。
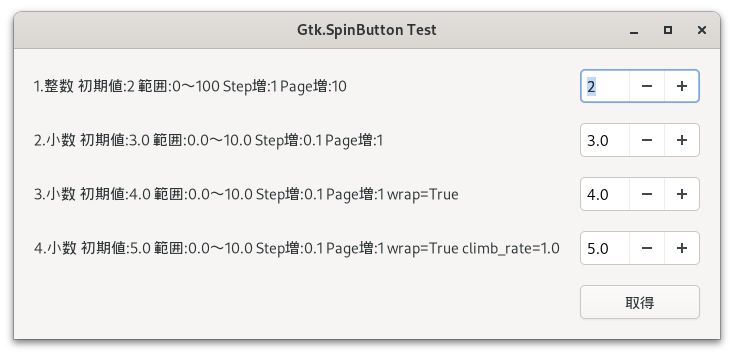
import gi
gi.require_version('Gtk', '4.0')
from gi.repository import Gtk
APPID = 'com.github.taniyoshima.g4_fblog_spinbutton'
class Gtk4TestTest(Gtk.Window):
def __init__(self, app):
Gtk.Window.__init__(
self, application=app, title='Gtk.SpinButton Test',
default_width=600, default_height=100)
grid = Gtk.Grid(
margin_top=20, margin_bottom=20,
margin_start=20, margin_end=20,
column_spacing=20, row_spacing=20
)
label1 = Gtk.Label(
label='1.整数 初期値:2 範囲:0〜100 Step増:1 Page増:10',
xalign=0.0
)
adjustment = Gtk.Adjustment(
lower=0, upper=100, value=2,
step_increment=1, page_increment=10
)
self.spinbutton1 = Gtk.SpinButton(
adjustment=adjustment
)
label2 = Gtk.Label(
label='2.小数 初期値:3.0 範囲:0.0〜10.0 Step増:0.1 Page増:1',
xalign=0.0
)
adjustment2 = Gtk.Adjustment(
lower=0.0, upper=10.0, value=3.0,
step_increment=0.1, page_increment=1
)
self.spinbutton2 = Gtk.SpinButton(
digits=1,
adjustment=adjustment2,
update_policy=Gtk.SpinButtonUpdatePolicy.IF_VALID
)
label3 = Gtk.Label(
label='3.小数 初期値:4.0 範囲:0.0〜10.0 Step増:0.1 Page増:1 wrap=True',
xalign=0.0
)
adjustment3 = Gtk.Adjustment(
lower=0.0, upper=10.0, value=4.0,
step_increment=0.1, page_increment=1
)
self.spinbutton3 = Gtk.SpinButton(
digits=1,
adjustment=adjustment3,
wrap=True
)
label4 = Gtk.Label(
label='4.小数 初期値:5.0 範囲:0.0〜10.0 '
'Step増:0.1 Page増:1 wrap=True climb_rate=1.0',
xalign=0.0
)
adjustment4 = Gtk.Adjustment(
lower=0.0, upper=10.0, value=5.0,
step_increment=0.1, page_increment=1
)
self.spinbutton4 = Gtk.SpinButton(
digits=1,
adjustment=adjustment4,
wrap=True,
climb_rate=1.0
)
button = Gtk.Button(label='取得')
button.connect('clicked', self.on_button_clicked)
grid.attach(label1, 0, 0, 1, 1)
grid.attach(self.spinbutton1, 1, 0, 1, 1)
grid.attach(label2, 0, 1, 1, 1)
grid.attach(self.spinbutton2, 1, 1, 1, 1)
grid.attach(label3, 0, 2, 1, 1)
grid.attach(self.spinbutton3, 1, 2, 1, 1)
grid.attach(label4, 0, 3, 1, 1)
grid.attach(self.spinbutton4, 1, 3, 1, 1)
grid.attach(button, 1, 4, 1, 1)
self.set_child(grid)
def on_button_clicked(self, button):
print('1.整数: ' + str(self.spinbutton1.get_value_as_int()))
print('2.小数: {:.1f}'.format(self.spinbutton2.get_value()))
print('3.小数: {:.1f}'.format(self.spinbutton3.get_value()))
print('4.小数: {:.1f}'.format(self.spinbutton4.get_value()))
class Gtk4TestApp(Gtk.Application):
def __init__(self):
Gtk.Application.__init__(self, application_id=APPID)
def do_activate(self):
window = Gtk4TestTest(self)
window.present()
def main():
app = Gtk4TestApp()
app.run()
if __name__ == '__main__':
main()
22〜80行目: Gtk.Label、Gtk.Adjustment、Gtk.SpinButtonの設定をしています。
97〜101行目: 82行目で定義したGtk.Buttonをクリックした場合に実行する関数
・4つのGtk.SpinButtonの値をターミナルに出力しています。
・整数はget_value_as_int()で取得した後、str()で文字列に変換しています。
・小数はget_value()で取得した後、{:.1f}.format()で小数点1位までの文字列にしています。
まとめ
- Gtk.SpinButtonに表示する値の範囲や値、増減量は、Gtk.Adjustmentで指定します。
- Gtk.SpinButtonの値は、メソッドget_value_as_int()(整数)やget_value()(小数)で取得します。
次回の予定
次回は、テキストを入力するのに使用するGtk.Entryについて見ていきます。