XMLデータにより定義したGtk.TextViewの使用
Gtk.TextView(以下、TextViewという)は、Gtk4で複数行のテキストを表示するためのWidgetです。このWidgetもXMLデータで作成することが可能であり、TextViewに表示する情報はPython側から編集することができます。
今回は、XMLデータで作成したTextViewにテキストを挿入したり、TextViewに表示データを編集する方法を紹介します。
今回紹介する内容
・ XMLデータで、Gtk.TreeViewを作成する。
・ TreeViewにテキストを挿入する。
・ TextViewに表示したテキストを取得する。
Gtk.TextViwの設定
XMLデータで、Gtk.TreeViewを作成する。
XMLデータでのGtk.TextViewの作成は、以下のようにおこないます。今回は、プロパティvexpandのみを指定しています。
<child>
<object class="GtkTextView" id="textview">
<property name="vexpand">True</property>
</object>
</child>
TreeViewにテキストを挿入する。
Gtk.TreeViewへのテキストの挿入は、以下の手順でおこないます。
1. Gtk.Bufferを作成する。
2. Gtk.Bufferにメソッドset_textでテキストを入れる。
set_textのパラメーター:
text: テキスト
length: テキストの文字数
3. Gtk.TextViewに、メソッドset_bufferによりGtk.Bufferをセットする。
@Gtk.Template.Callback()
def on_button1_clicked(self, button):
buffer = Gtk.TextBuffer()
buffer.set_text("Hello\nGood-Bye", 14)
self.textview.set_buffer(buffer)
TextViewに表示したテキストを取得する。
Gtk.TextViweからのテキストの取得は、以下の手順でおこないます。
1. Gtk.TextViewからメソッドget_bufferでGtk.TextBufferを取得する。
2. 取得したGtk.TextBufferからメソッドget_textにより、テキストを取得する。
get_textのパラメーター:
start(Gtk.TextIter): 取得するテキストの最初の位置
end(Gtk.TextIter): 取得するテキストの最後の位置
include_hidden_chars(bool型): 非表示のテキストを含めるかどうか
start及びendはメソッドget_start_iter()とget_end_iter()を使用することで、
Gtk.TextBuffer(Gtk.TextViewより取得)より取得する。
@Gtk.Template.Callback()
def on_button2_clicked(self, button):
text = self.textview.get_buffer().get_text(
self.textview.get_buffer().get_start_iter(),
self.textview.get_buffer().get_end_iter(),
False
)
print(text)
サンプルプログラム
以下のサンプルプログラムを実行すると図のようなGtk.TextViewの2つのボタン(ラベルが「書き込み」と「取得」)が表示されます。
「書き込み」ボタンを押すと、Gtk.TextViewに指定したテキストが表示されます。また、「取得」ボタンを押すと、Gtk.TextView上のテキストを取得してターミナルに表示します。
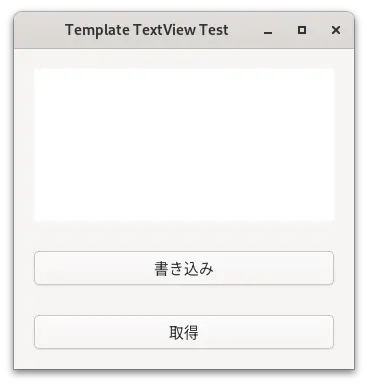
実行方法
下の2つのファイルを同じフォルダに保存して、そのフォルダで以下のコマンドを実行します。
python main.py
<?xml version="1.0" encoding="UTF-8"?>
<interface>
<template class="window" parent="GtkWindow">
<property name="title">Template TextView Test</property>
<child>
<object class="GtkBox" id="box">
<property name="width_request">300</property>
<property name="height_request">280</property>
<property name="margin_top">20</property>
<property name="margin_bottom">20</property>
<property name="margin_start">20</property>
<property name="margin_end">20</property>
<property name="spacing">30</property>
<property name="orientation">vertical</property>
<child>
<object class="GtkTextView" id="textview">
<property name="vexpand">True</property>
</object>
</child>
<child>
<object class="GtkButton" id="button1">
<property name="label">書き込み</property>
<signal name="clicked" handler="on_button1_clicked"/>
</object>
</child>
<child>
<object class="GtkButton" id="button2">
<property name="label">取得</property>
<signal name="clicked" handler="on_button2_clicked"/>
</object>
</child>
</object>
</child>
</template>
</interface>
import os
import gi
gi.require_version('Gtk', '4.0')
from gi.repository import Gtk
APPID = 'com.github.taniyoshima.g4_fblogt_textview'
@Gtk.Template(filename=os.path.dirname(__file__) + '/ui_file.ui')
class Gtk4TestTest(Gtk.Window):
__gtype_name__ = "window"
textview = Gtk.Template.Child()
def __init__(self, app):
Gtk.Window.__init__(
self, application=app)
@Gtk.Template.Callback()
def on_button1_clicked(self, button):
buffer = Gtk.TextBuffer()
buffer.set_text("Hello\nGood-Bye", 14)
self.textview.set_buffer(buffer)
@Gtk.Template.Callback()
def on_button2_clicked(self, button):
text = self.textview.get_buffer().get_text(
self.textview.get_buffer().get_start_iter(),
self.textview.get_buffer().get_end_iter(),
False
)
print(text)
class Gtk4TestApp(Gtk.Application):
def __init__(self):
Gtk.Application.__init__(self, application_id=APPID)
def do_activate(self):
window = Gtk4TestTest(self)
window.present()
def main():
app = Gtk4TestApp()
app.run()
if __name__ == '__main__':
main()